Python
If you want to use the open source SEGY libraries such as segyio, segypy, or segpy then I would recommend that you install Python 3.6.1 as all these libraries are compatible with this version. By using these libraries managing SEGY files with Python becomes incredibly easy. Pretty much all the functionality that is available is SEGY tools such as Seismic Unix can now be available simply by writing a Python script and importing the relevant modules.
Many people have a natural concern about using Python because in order to use Python scripts Python needs to be installed on their machine. As most of the people I work with use Windows this concern can be legitimate particularly if administrator privileges are required (IT departments tend not to understand Python and how it works).
By far the easiest way to install Python on Windows is to do it for the currently installed user. This requires no administrative privileges and also means that any libraries that are subsequently required can be installed with a few keys strokes in the command window.
To install Python on Windows, download Python 3.6.1 from here and select the Windows x86-64 executable installer,
Installing Python
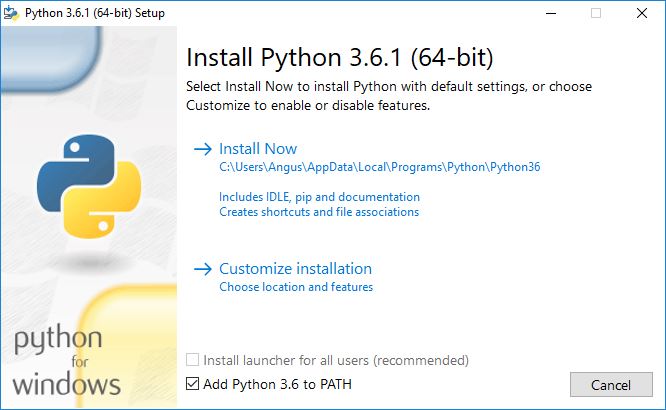
Run the Python 3.6.1 installer. Use the default settings and ensure that the “Add Python 3,6 to PATH” checkbox is checked. Then click on the “Install Now” link in the upper part of the window.
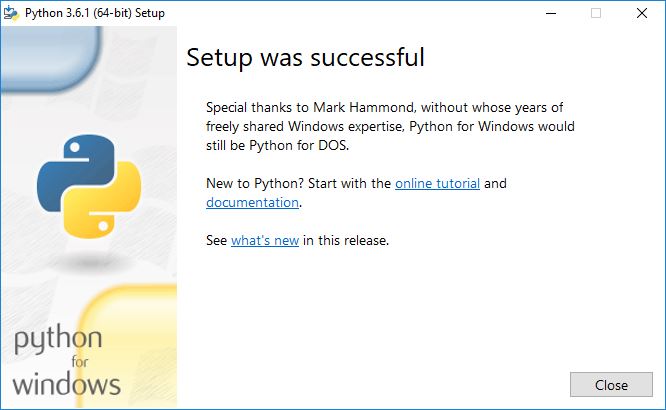
If all goes well then Python 3.6.1 will be installed in the following path:
C:\Users\”Your user name”\AppData\Local\Programs\Python\Python36
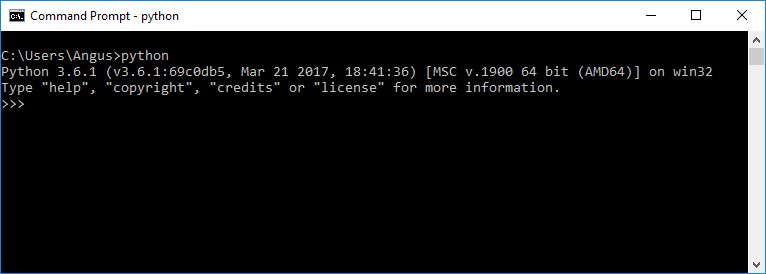
Open a command prompt and type python, You should get a python shell that looks something like this:
Python 3.6.1 (v3.6.1:69c0db5, Mar 21 2017, 18:41:36) [MSC v.1900 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>>
Type Ctrl+Z and and hit return, you will exit the shell.
Installing Libraries
Now that Python is installed you can install any libraries that you may need from the command line. To install the Gooey argument parser (GUI front end) type the following:
pip install Gooey
This will try to install the Gooey front end argument parser. The following output to the console is typical:
Collecting Gooey
Using cached https://files.pythonhosted.org/packages/37/2a/f173ab639dd06531c7e3082011adeb77c876fb7095ca94f08956dd3daf79/Gooey-1.0.2-py2.py3-none-any.whl
Requirement already satisfied: Pillow>=4.3.0 in c:\users\angus\appdata\local\programs\python\python36\lib\site-packages (from Gooey) (5.4.1)
Requirement already satisfied: psutil>=5.4.2 in c:\users\angus\appdata\local\programs\python\python36\lib\site-packages (from Gooey) (5.6.1)
Requirement already satisfied: wxpython>=4.0.0b1 in c:\users\angus\appdata\local\programs\python\python36\lib\site-packages (from Gooey) (4.0.4)
Requirement already satisfied: six in c:\users\angus\appdata\local\programs\python\python36\lib\site-packages (from wxpython>=4.0.0b1->Gooey) (1.12.0)
Installing collected packages: Gooey
Successfully installed Gooey-1.0.2
To install other libraries or modules the syntax is similar:
pip install "the library you want to install"
Segyio
Segyio is a small LGPL licensed C library for easy interaction with SEG Y formatted seismic data, with language bindings for Python and Matlab. Segyio is an attempt to create an easy-to-use, embeddable, community-oriented library for seismic applications.
Feature summary
- A low-level C interface with few assumptions; easy to bind to other languages
- Read and write binary and textual headers
- Read and write traces and trace headers
- Simple, powerful, and native-feeling Python interface with numpy integration
- xarray integration with netcdf_segy
- Some simple applications with unix philosophy
Segypy
Segypy is a Python module for reading/writing of SEG-Y formatted files. As it stands on GitHub segypy has a significant limitation, that is clearly outlined in the documentation, namely:
“Currently you can READ IBM Floats, IEEE, 1, 2 and 4 byte INTEGER formatted data, and WRITE anything but IBM Floats. “
Writting IBM floats is quite a useful thing to do in the offshore survey sector where many clients expect SEGY files to have this form of encoding. To resolve this problem I have written a patch for this module (library) that allows reading and writting to or from any format specified in the SEGY standard (rev 1) format. If you want to make use of this patch then please contact me.
SegPy
Segpy is open source software created by Sixty North and licensed under the GNU Affero General Public License. This library is extremely well written and contains loads of useful utilities.
The beauty of segpy is the code readability. This library is a great companion when writting scripts using either segyio or segypy particularly as its interpretation of the standard is robust.